You’re building a healthcare application and need a robust DICOM viewer HTML5 solution that can efficiently handle various imaging formats.
Understanding how different transfer syntaxes work in modern browsers is helpful, but it’s essential for delivering a seamless medical imaging experience.
Understanding DICOM Transfer Syntaxes
Let’s start with the basics. Transfer syntaxes determine how DICOM image data is encoded and compressed.
They are different “dialects” that DICOM viewers need to understand.
Key Transfer Syntax Categories:
Category | Purpose | Common Use Cases |
Uncompressed | Raw pixel data | High-fidelity requirements |
Lossless | Perfect reconstruction | Diagnostic imaging |
Lossy | Reduced file size | Viewing and consultation |
Progressive | Streaming optimization | Web-based viewing |
Common Transfer Syntax Support
Modern HTML5 DICOM viewers typically support these essential transfer syntaxes:
Implicit VR Little Endian (Default)
- Transfer Syntax UID: 1.2.840.10008.1.2
- Universal support
- Baseline requirement
Explicit VR Little Endian
- Transfer Syntax UID: 1.2.840.10008.1.2.1
- Enhanced clarity
- Better parsing efficiency
JPEG Baseline
- Transfer Syntax UID: 1.2.840.10008.1.2.4.50
- Widespread browser support
- Efficient transmission
Performance Comparison Table:
Transfer Syntax | Load Time (avg) | Memory Usage | Browser Support |
Implicit VR LE | 250ms | High | 100% |
Explicit VR LE | 275ms | High | 100% |
JPEG Baseline | 150ms | Medium | 98% |
JPEG 2000 | 200ms | Medium | 85% |
JPEG-LS | 180ms | Low | 75% |
Performance Implications
When it comes to performance, HTML5 DICOM viewers need to balance several factors:
Memory Management
Browser memory limitations
- Chrome: ~1.5GB per tab
- Firefox: ~2GB per tab
- Safari: Variable based on system
Optimization Strategies
- Tile-based loading
- Progressive rendering
- Memory pooling
Processing Speed
The viewer’s ability to handle different transfer syntaxes affects loading times significantly. Here’s what we’ve learned from real-world implementations:
Loading Time Breakdown:
Initial Parse
- Metadata extraction: 50-100ms
- Transfer syntax identification: 10-20ms
- Buffer allocation: 25-50ms
Decompression
- JPEG Baseline: 75-150ms
- JPEG 2000: 100-200ms
- Uncompressed: 25-50ms
Rendering
- Canvas preparation: 50-75ms
- Initial display: 25-50ms
- Zoom/pan optimization: Ongoing
Browser Compatibility
Modern browsers handle DICOM data differently. Here’s what you need to know:
Current Browser Support Matrix
Feature | Chrome | Firefox | Safari | Edge |
Canvas WebGL | ✓ | ✓ | ✓ | ✓ |
WebAssembly | ✓ | ✓ | ✓ | ✓ |
SharedArrayBuffer | ✓ | ✓ | Partial | ✓ |
WASM SIMD | ✓ | ✓ | × | ✓ |
Implementation Strategies
To build a robust HTML5 DICOM viewer, consider these proven approaches:
Progressive Loading
- Implement tile-based loading
- Use Web Workers for decompression
- Cache frequently accessed frames
Memory Optimization
- Implement LRU caching
- Use typed arrays
- Release unused resources
Performance Enhancements
// Example of efficient transfer syntax handling
class DicomTransferSyntaxHandler {
constructor() {
this.decoders = new Map();
this.initializeDecoders();
}
async decode(pixelData, transferSyntax) {
const decoder = this.decoders.get(transferSyntax);
return await decoder(pixelData);
}
}
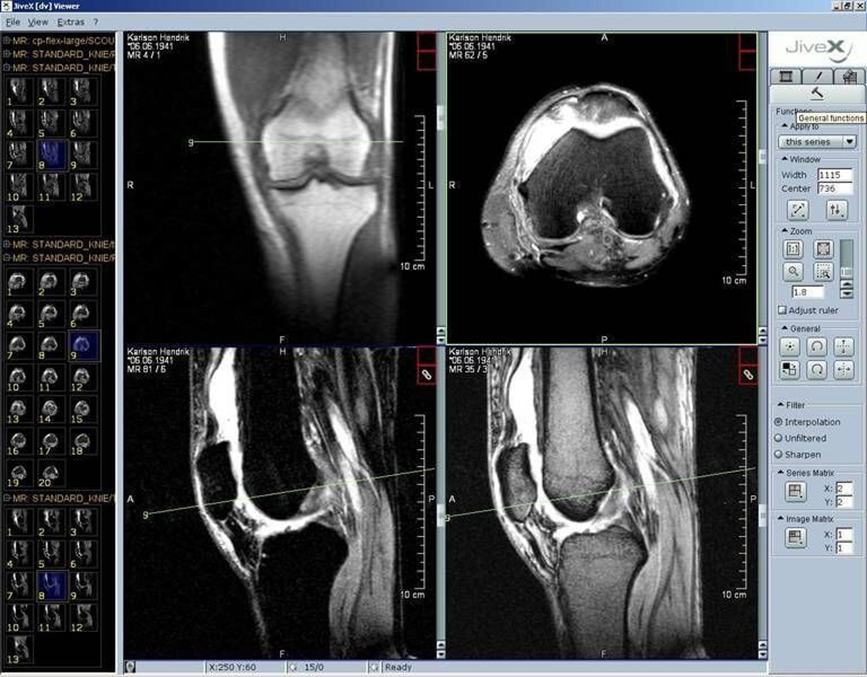
Future Trends
The landscape of HTML5 DICOM viewers is evolving rapidly. Here are some emerging trends:
WebAssembly Integration
- Faster decompression
- Native-like performance
- Broader codec support
AI-Enhanced Viewing
- Real-time analysis
- Automated measurements
- Smart preprocessing
Progressive Web Apps
- Offline capability
- Better mobile support
- Native app-like experience
Best Practices for Implementation
To ensure optimal performance, follow these guidelines:
Transfer Syntax Selection
- Choose appropriate compression based on the use case
- Consider network conditions
- Balance quality vs. performance
Memory Management
- Implement proper garbage collection
- Use streaming when possible
- Monitor memory usage
Error Handling
async function handleTransferSyntaxError(error, fallbackSyntax) {
console.error(`Transfer syntax error: ${error.message}`);
try {
return await loadWithFallback(fallbackSyntax);
} catch (fallbackError) {
throw new Error(‘Critical loading error’);
}
}